Front-end Engineer Technical Interview - Conceptual questions
- 11 senior front-end developer interview questions and answers
- 2023's Top 5 Senior Frontend Engineer Interview Questions
Front-end Engineer Technical Interview - Coding
- GreatFrontEnd - Front End Interview Questions - By ex-FAANG interviewers
- Interview Kickstart - Front-End Interview Prep
- InterviewBit - Front-End Developer Interview Questions

1. Reverse String using inbuilt methods and without methods

2. Sort the number array in descending order
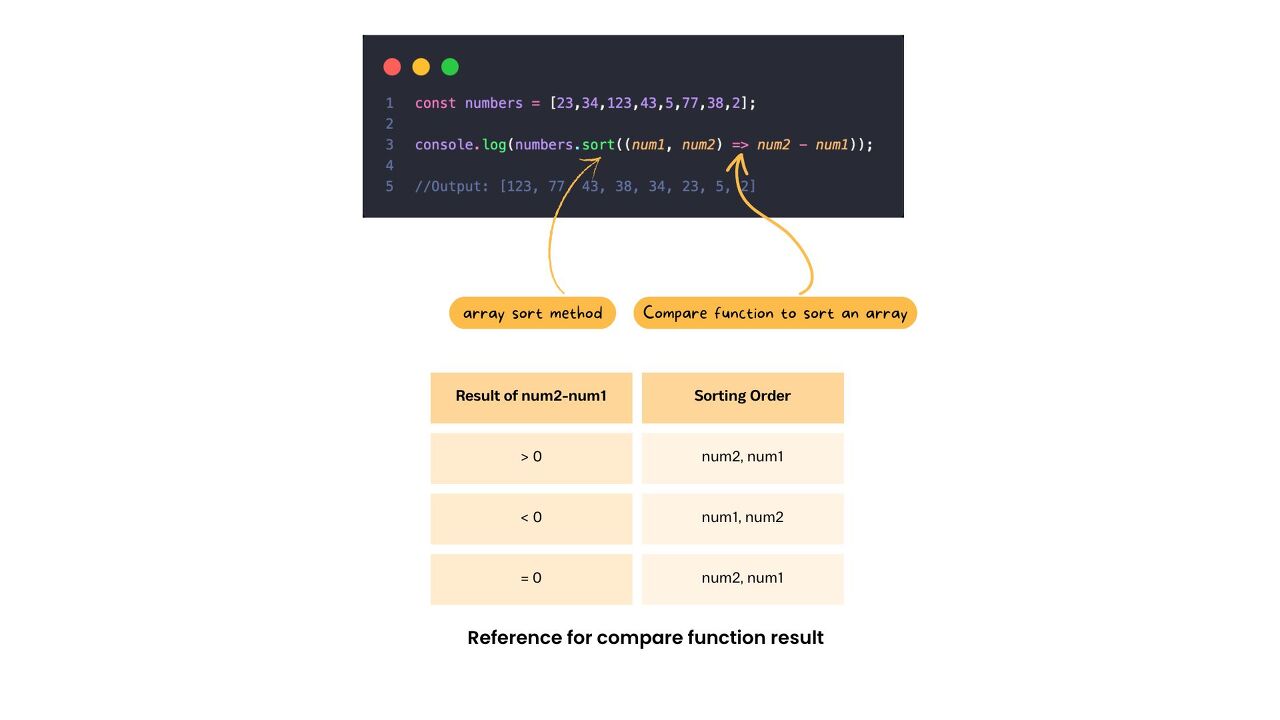
The sort method sorts data in ascending order according to Unicode value.
If you want to customize the sorting order, you will need to use a compare function, as shown in the example.
MINI PROJECTS
- 16 front-end projects (with designs) to help improve your coding skills
- Frontend Mentor - challenges
Javascript
Javascript tutorial - Dom Object Model
Q. Optimization
How to optimize our front-end page?
- Improve Server Response: Enhance the server's performance to reduce the time it takes to deliver resources to the client. This can be achieved through various techniques such as caching, compression, and optimizing database queries.
- Use External CSS and JavaScript: Instead of using inline or internal styles and scripts, separate them into external files. This allows the browser to cache and load these resources separately, improving page load speed and reducing redundancy.
- Utilize Frameworks: Employ front-end frameworks like React, Angular, or Vue.js to create responsive and efficient user interfaces. These frameworks provide pre-built components, state management, and performance optimizations out of the box.
- Leverage Open-Source Libraries: Use open-source libraries like Bootstrap or Material-UI to handle browser-specific styling issues. These libraries offer pre-styled components and ensure cross-browser compatibility, saving development time and effort.
- Implement Progressive Loading: Implement techniques like Lazy Loading to optimize the rendering of heavy elements, such as images or videos. Lazy Loading defers the loading of non-visible content until the user interacts with it, reducing the initial load time.
- Optimize Resource Placement: Connect your stylesheet in the <head> section of the HTML document and scripts at the top of the <body> tag. This allows the styles to be applied early and scripts to be loaded before rendering, improving the overall performance.
- Use Browser Storage: Utilize browser storage mechanisms like localStorage or sessionStorage to store user-specific private data. This reduces the need to fetch data from the server repeatedly, improving performance and providing a better user experience.
By implementing these optimizations, you can reduce resource consumption, improve page load speed, enhance responsiveness, and deliver a more efficient and user-friendly front-end experience.
Free Web Development Courses
Here are the Free but Best Web Development Courses along with the study plan.
Phase 1: Foundations for HTML, CSS, and Javascript (Days 1-14)
- Day 1-3: Introduction to HTML
- Study Materials:
- FreeCodeCamp HTML Crash Course (https://www.youtube.com/watch?v=UB1O30fR-EE)
- Practice creating simple web pages using HTML
- Study Materials:
- Day 4-6: Introduction to CSS
- Study Materials:
- YouTube Channel: The Net Ninja (https://www.youtube.com/c/TheNetNinja)
- CSS Crash Course for Absolute Beginners (https://www.youtube.com/watch?v=yfoY53QXEnI)
- Experiment with styling HTML elements using CSS
If you want an Udemy course for HTML & CSS, recommend Modern HTML & CSS From The Beginning (Including Sass) by Brad Traversy
- Study Materials:
- Day 7-9: Introduction to JavaScript
- Study Materials:
- YouTube Channel: freeCodeCamp.org (https://www.youtube.com/c/Freecodecamp)
- Javascript Full Course for free - Bro Code /8 hours(https://www.youtube.com/watch?v=8dWL3wF_OMw)
- Javascript Crash course for beginners and project playlist
- Javascript DOM Crash Course – freecodecamp (2.4 hours)
- JS OOP Crash Course
- High Order Functions & Arrays Method
- Async JS Crash Course – Callbacks, Promises, Async Await
- AJAX Crash course
- Fetch API
- How to use Fetch API in 6 Minutes
- Framework crash course
- Zero to Mastery - complete web developer - HTTPS/JSON/AJAX + Asynchronous javascript
- Study Materials:
- Day 10-14: Responsive Web Design
- Study Materials:
- YouTube Channel: DesignCourse (https://www.youtube.com/c/DesignCourse)
- Responsive Web Design Tutorial for Beginners (https://www.youtube.com/watch?v=2KL-z9A56SQ)
- Study CSS media queries
- Learn techniques for creating mobile-friendly websites
- Implement responsive designs using CSS frameworks like Bootstrap or Foundation
- Study Materials:
Phase 2: Front-End Development (Days 15-42)
- Day 15-21: Advanced HTML and CSS
- Study Materials:
- YouTube Channel: Kevin Powell (https://www.youtube.com/kepowob)
- HTML & CSS Crash Course (https://www.youtube.com/watch?v=D-h8L5hgW-w)
- Dive deeper into HTML5 features (semantic elements, forms, video/audio)
- Explore CSS3 properties and animations
- Study Materials:
- Day 22-28: JavaScript and DOM Manipulation
- Study Materials:
- YouTube Channel: Academind (https://www.youtube.com/c/Academind)
- JavaScript DOM Crash Course (https://www.youtube.com/watch?v=0ik6X4DJKCc)
- Learn advanced JavaScript concepts (objects, arrays, JSON)
- Understand the Document Object Model (DOM) and its manipulation using JavaScript
- Practice building interactive web pages
- Study Materials:
- Day 29-35: Front-End Frameworks
- Study Materials:
- YouTube Channel: The Net Ninja (https://www.youtube.com/c/TheNetNinja)
- React Tutorial for Beginners (https://www.youtube.com/watch?v=O6P86uwfdR0)
- Angular Crash Course (https://www.youtube.com/watch?v=_TLhUCjY9iA)
- Vue.js Crash Course (https://www.youtube.com/watch?v=4deVCNJq3qc)
- Choose a front-end framework (e.g., React, Angular, Vue.js)
- Learn the framework’s basics, including components and state management
- Build a small project using the chosen framework
- Study Materials:
- Day 36-42: Version Control and Collaboration
- Study Materials:
- YouTube Channel: Corey Schafer (https://www.youtube.com/c/Coreyms)
- Git & GitHub Crash Course (https://www.youtube.com/watch?v=SWYqp7iY_Tc)
- Learn Git for version control
- Understand branching, merging, and resolving conflicts
- Collaborate on a project using Git and platforms like GitHub or GitLab
- Study Materials:
Phase 3: Back-End Development (Days 43-70)
- Day 43-49: Server-Side Programming
- Study Materials:
- YouTube Channel: The Net Ninja (https://www.youtube.com/c/TheNetNinja)
- Node.js Crash Course (https://www.youtube.com/watch?v=fBNz5xF-Kx4)
- Python Django Crash Course (https://www.youtube.com/watch?v=D6esTdOLXh4)
- Choose a server-side language (e.g., Node.js, Python)
- Learn the basics of the chosen language
- Build a simple server-side application
- Study Materials:
- Day 50-56: Databases and Data Storage
- Study Materials:
- YouTube Channel: Traversy Media (https://www.youtube.com/c/TraversyMedia)
- MySQL Crash Course (https://www.youtube.com/watch?v=9ylj9NR0Lcg)
- MongoDB Crash Course (https://www.youtube.com/watch?v=-56x56UppqQ)
- Study SQL and relational databases
- Learn how to interact with databases using your chosen language
- Build a database-driven web application
- Study Materials:
- Day 57-63: RESTful APIs and Integration
- Study Materials:
- YouTube Channel: Traversy Media (https://www.youtube.com/c/TraversyMedia)
- REST API Crash Course (https://www.youtube.com/watch?v=5GcQtLDGXy8)
- Understand REST architecture and principles
- Learn how to build and consume APIs
- Integrate your front-end and back-end applications
- Study Materials:
- Day 64-70: Security and Authentication
- Study Materials:
- YouTube Channel: Web Dev Simplified (https://www.youtube.com/c/WebDevSimplified)
- Web Security Fundamentals (https://www.youtube.com/watch?v=UKcTLVrXQ7Q)
- Explore web application security best practices
- Learn about authentication and authorization methods
- Implement user authentication in your web application
- Study Materials:
Phase 4: Project Development (Days 71-90)
- Day 71-80: Full-Stack Project
- Identify a project idea
- Plan and design the project’s architecture
- Develop the project using your chosen technologies
- Test and debug the application
- Day 81-90: Deployment and Optimization
- Study Materials:
- YouTube Channel: Traversy Media (https://www.youtube.com/c/TraversyMedia)
- Deploying a Website Crash Course (https://www.youtube.com/watch?v=yoXxQCseS4c)
- Deploy your project to a web server or cloud platform
- Optimize the performance of your application
- Implement caching, minification, and other optimization techniques
- Perform testing and gather feedback for further improvement
- Study Materials:
댓글